イントロダクションIntroduction
Laravel Promptsは、美しくユーザーフレンドリーなUIをコマンドラインアプリケーションに追加するためのPHPパッケージで、プレースホルダテキストやバリデーションなどのブラウザにあるような機能を備えています。Laravel Prompts[https://github.com/laravel/prompts] is a PHP package for adding beautiful and user-friendly forms to your command-line applications, with browser-like features including placeholder text and validation.
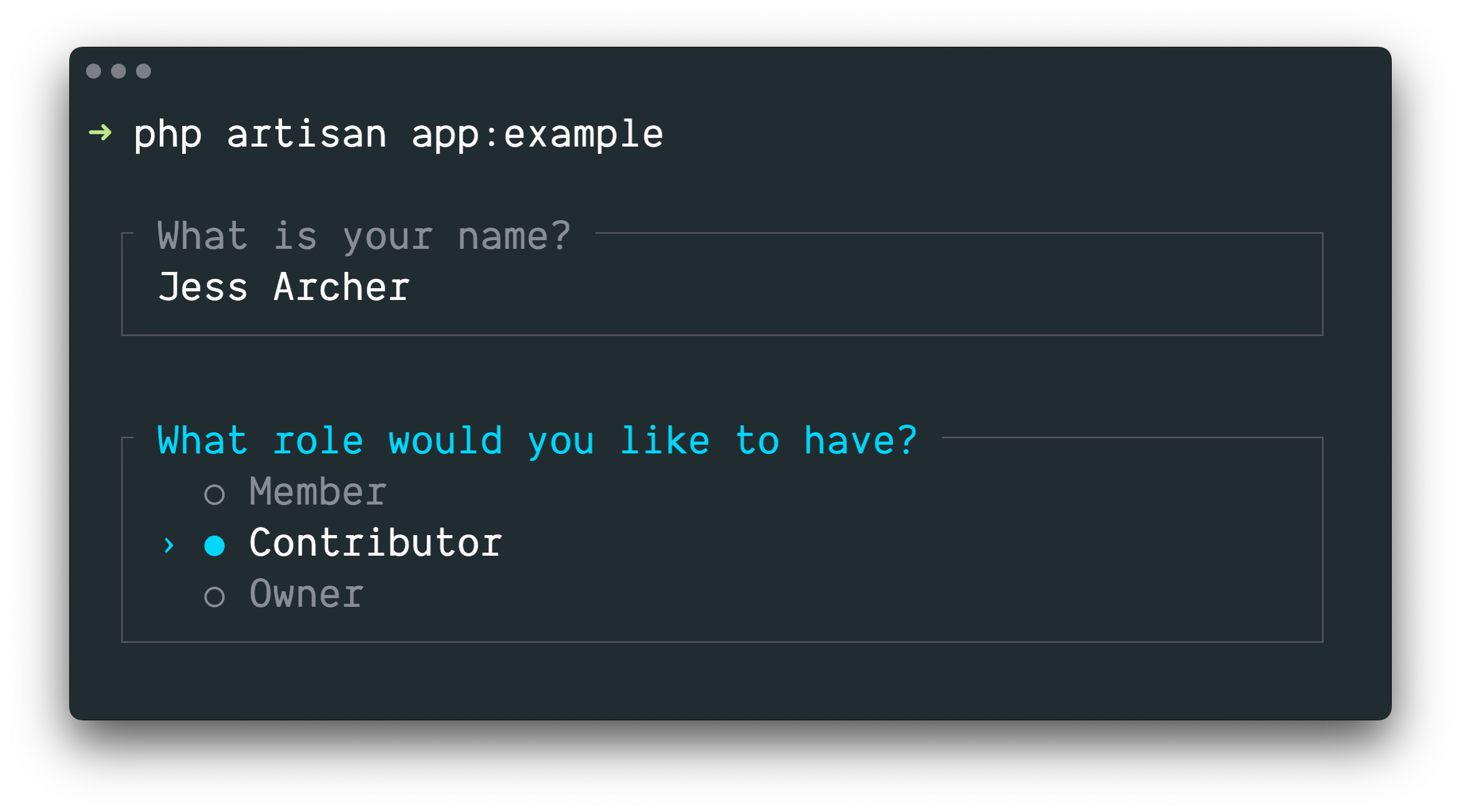
Laravel Promptsは、Artisanコンソールコマンドでユーザー入力を受けるために最適ですが、コマンドラインのPHPプロジェクトでも使用できます。Laravel Prompts is perfect for accepting user input in your Artisan console commands[/docs/{{version}}/artisan#writing-commands], but it may also be used in any command-line PHP project.
未サポートの環境とフォールバックのドキュメントをご覧ください。[!NOTE]
Note: Laravel PromptsはmacOS、Linux、WindowsのWSLをサポートしています。詳しくは、
Laravel Prompts supports macOS, Linux, and Windows with WSL. For more information, please see our documentation on unsupported environments & fallbacks[#fallbacks].
インストールInstallation
Laravelの最新リリースには、はじめからLaravel Promptsを用意してあります。Laravel Prompts is already included with the latest release of Laravel.
Composerパッケージマネージャを使用して、他のPHPプロジェクトにLaravel Promptsをインストールすることもできます。Laravel Prompts may also be installed in your other PHP projects by using the Composer package manager:
composer require laravel/prompts
利用可能なプロンプトAvailable Prompts
テキストText
text
関数は、指定した質問でユーザーに入力を促し、回答を受け、それを返します。The text
function will prompt the user with the given question, accept their input, and then return it:
use function Laravel\Prompts\text;
$name = text('What is your name?');
プレースホルダテキストとデフォルト値、ヒントの情報も指定できます。You may also include placeholder text, a default value, and an informational hint:
$name = text(
label: 'What is your name?',
placeholder: 'E.g. Taylor Otwell',
default: $user?->name,
hint: 'This will be displayed on your profile.'
);
必須値Required Values
入力値が必須の場合は、required
引数を渡してください。If you require a value to be entered, you may pass the required
argument:
$name = text(
label: 'What is your name?',
required: true
);
バリデーション・メッセージをカスタマイズしたい場合は、文字列を渡すこともできます。If you would like to customize the validation message, you may also pass a string:
$name = text(
label: 'What is your name?',
required: 'Your name is required.'
);
追加のバリデーションAdditional Validation
最後に、追加のバリデーションロジックを実行したい場合は、validate
引数にクロージャを渡します。Finally, if you would like to perform additional validation logic, you may pass a closure to the validate
argument:
$name = text(
label: 'What is your name?',
validate: fn (string $value) => match (true) {
strlen($value) < 3 => 'The name must be at least 3 characters.',
strlen($value) > 255 => 'The name must not exceed 255 characters.',
default => null
}
);
クロージャは入力された値を受け取り、エラーメッセージを返すか、バリデーションに合格した場合は、null
を返します。The closure will receive the value that has been entered and may return an error message, or null
if the validation passes.
パスワードPassword
password
関数はtext
関数と似ていますが、コンソールで入力されるユーザー入力をマスクします。これはパスワードのような機密情報を要求するときに便利です:The password
function is similar to the text
function, but the user's input will be masked as they type in the console. This is useful when asking for sensitive information such as passwords:
use function Laravel\Prompts\password;
$password = password('What is your password?');
プレースホルダテキストと情報のヒントも含められます。You may also include placeholder text and an informational hint:
$password = password(
label: 'What is your password?',
placeholder: 'password',
hint: 'Minimum 8 characters.'
);
必須値Required Values
入力値が必須の場合は、required
引数を渡してください。If you require a value to be entered, you may pass the required
argument:
$password = password(
label: 'What is your password?',
required: true
);
バリデーション・メッセージをカスタマイズしたい場合は、文字列を渡すこともできます。If you would like to customize the validation message, you may also pass a string:
$password = password(
label: 'What is your password?',
required: 'The password is required.'
);
追加のバリデーションAdditional Validation
最後に、追加のバリデーションロジックを実行したい場合は、validate
引数にクロージャを渡します。Finally, if you would like to perform additional validation logic, you may pass a closure to the validate
argument:
$password = password(
label: 'What is your password?',
validate: fn (string $value) => match (true) {
strlen($value) < 8 => 'The password must be at least 8 characters.',
default => null
}
);
クロージャは入力された値を受け取り、エラーメッセージを返すか、バリデーションに合格した場合は、null
を返します。The closure will receive the value that has been entered and may return an error message, or null
if the validation passes.
確認Confirm
ユーザーに "yes/no"の確認を求める必要がある場合は、confirm
関数を使います。ユーザーは矢印キーを使うか、y
またはn
を押して回答を選択します。この関数はtrue
またはfalse
を返します。If you need to ask the user for a "yes or no" confirmation, you may use the confirm
function. Users may use the arrow keys or press y
or n
to select their response. This function will return either true
or false
.
use function Laravel\Prompts\confirm;
$confirmed = confirm('Do you accept the terms?');
また、デフォルト値、「はい」/「いいえ」ラベルをカスタマイズする文言、情報ヒントも含められます。You may also include a default value, customized wording for the "Yes" and "No" labels, and an informational hint:
$confirmed = confirm(
label: 'Do you accept the terms?',
default: false,
yes: 'I accept',
no: 'I decline',
hint: 'The terms must be accepted to continue.'
);
必須の"Yes"Requiring "Yes"
必要であれば、required
引数を渡し、ユーザーに"Yes"を選択させることもできます。If necessary, you may require your users to select "Yes" by passing the required
argument:
$confirmed = confirm(
label: 'Do you accept the terms?',
required: true
);
バリデーション・メッセージをカスタマイズしたい場合は、文字列を渡すこともできます。If you would like to customize the validation message, you may also pass a string:
$confirmed = confirm(
label: 'Do you accept the terms?',
required: 'You must accept the terms to continue.'
);
選択Select
ユーザーにあらかじめ定義された選択肢から選ばせる必要がある場合は、select
関数を使います。If you need the user to select from a predefined set of choices, you may use the select
function:
use function Laravel\Prompts\select;
$role = select(
'What role should the user have?',
['Member', 'Contributor', 'Owner'],
);
デフォルト選択肢と情報のヒントも指定できます。You may also specify the default choice and an informational hint:
$role = select(
label: 'What role should the user have?',
options: ['Member', 'Contributor', 'Owner'],
default: 'Owner',
hint: 'The role may be changed at any time.'
);
options
引数に連想配列を渡し、選択値の代わりにキーを返すこともできます。You may also pass an associative array to the options
argument to have the selected key returned instead of its value:
$role = select(
label: 'What role should the user have?',
options: [
'member' => 'Member',
'contributor' => 'Contributor',
'owner' => 'Owner'
],
default: 'owner'
);
5選択肢を超えると、選択肢がリスト表示されます。scroll
引数を渡し、カスタマイズ可能です。Up to five options will be displayed before the list begins to scroll. You may customize this by passing the scroll
argument:
$role = select(
label: 'Which category would you like to assign?',
options: Category::pluck('name', 'id'),
scroll: 10
);
バリデーションValidation
他のプロンプト関数とは異なり、他に何も選択できなくなるため、select
関数はrequired
引数を受けません。しかし、選択肢を提示したいが、選択されないようにする必要がある場合は、validate
引数にクロージャを渡してください。Unlike other prompt functions, the select
function doesn't accept the required
argument because it is not possible to select nothing. However, you may pass a closure to the validate
argument if you need to present an option but prevent it from being selected:
$role = select(
label: 'What role should the user have?',
options: [
'member' => 'Member',
'contributor' => 'Contributor',
'owner' => 'Owner'
],
validate: fn (string $value) =>
$value === 'owner' && User::where('role', 'owner')->exists()
? 'An owner already exists.'
: null
);
options
引数が連想配列の場合、クロージャは選択されたキーを受け取ります。連想配列でない場合は選択された値を受け取ります。クロージャはエラーメッセージを返すか、バリデーションに成功した場合はnull
を返してください。If the options
argument is an associative array, then the closure will receive the selected key, otherwise it will receive the selected value. The closure may return an error message, or null
if the validation passes.
複数選択Multi-select
ユーザーが複数の選択肢を選択できるようにする必要がある場合は、multiselect
関数を使用します。If you need to the user to be able to select multiple options, you may use the multiselect
function:
use function Laravel\Prompts\multiselect;
$permissions = multiselect(
'What permissions should be assigned?',
['Read', 'Create', 'Update', 'Delete']
);
デフォルト選択肢と情報のヒントも指定できます。You may also specify default choices and an informational hint:
use function Laravel\Prompts\multiselect;
$permissions = multiselect(
label: 'What permissions should be assigned?',
options: ['Read', 'Create', 'Update', 'Delete'],
default: ['Read', 'Create'],
hint: 'Permissions may be updated at any time.'
);
options
引数に連想配列を渡し、選択値の代わりにキーを返させることもできます。You may also pass an associative array to the options
argument to return the selected options' keys instead of their values:
$permissions = multiselect(
label: 'What permissions should be assigned?',
options: [
'read' => 'Read',
'create' => 'Create',
'update' => 'Update',
'delete' => 'Delete'
],
default: ['read', 'create']
);
5選択肢を超えると、選択肢がリスト表示されます。scroll
引数を渡し、カスタマイズ可能です。Up to five options will be displayed before the list begins to scroll. You may customize this by passing the scroll
argument:
$categories = multiselect(
label: 'What categories should be assigned?',
options: Category::pluck('name', 'id'),
scroll: 10
);
必須値Requiring a Value
デフォルトで、ユーザーは0個以上の選択肢を選択できます。required
引数を渡せば、代わりに1つ以上の選択肢を強制できます。By default, the user may select zero or more options. You may pass the required
argument to enforce one or more options instead:
$categories = multiselect(
label: 'What categories should be assigned?',
options: Category::pluck('name', 'id'),
required: true,
);
バリデーションメッセージをカスタマイズしたい場合は、required
引数に文字列を指定します。If you would like to customize the validation message, you may provide a string to the required
argument:
$categories = multiselect(
label: 'What categories should be assigned?',
options: Category::pluck('name', 'id'),
required: 'You must select at least one category',
);
バリデーションValidation
選択肢を提示するが、その選択肢が選択されないようにする必要がある場合は、validate
引数にクロージャを渡してください。You may pass a closure to the validate
argument if you need to present an option but prevent it from being selected:
$permissions = multiselect(
label: 'What permissions should the user have?',
options: [
'read' => 'Read',
'create' => 'Create',
'update' => 'Update',
'delete' => 'Delete'
],
validate: fn (array $values) => ! in_array('read', $values)
? 'All users require the read permission.'
: null
);
options
引数が連想配列の場合、クロージャは選択されたキーを受け取ります。連想配列でない場合は選択された値を受け取ります。クロージャはエラーメッセージを返すか、バリデーションに成功した場合はnull
を返してください。If the options
argument is an associative array then the closure will receive the selected keys, otherwise it will receive the selected values. The closure may return an error message, or null
if the validation passes.
候補Suggest
suggest
関数を使用すると、可能性のある選択肢を自動補完できます。ユーザーは自動補完のヒントに関係なく、任意の答えを入力することもできます。The suggest
function can be used to provide auto-completion for possible choices. The user can still provide any answer, regardless of the auto-completion hints:
use function Laravel\Prompts\suggest;
$name = suggest('What is your name?', ['Taylor', 'Dayle']);
あるいは、suggest
関数の第2引数にクロージャを渡すこともできます。クロージャはユーザーが入力文字をタイプするたびに呼び出されます。クロージャは文字列パラメータにこれまでのユーザー入力を受け取り、オートコンプリート用の選択肢配列を返す必要があります。Alternatively, you may pass a closure as the second argument to the suggest
function. The closure will be called each time the user types an input character. The closure should accept a string parameter containing the user's input so far and return an array of options for auto-completion:
$name = suggest(
'What is your name?',
fn ($value) => collect(['Taylor', 'Dayle'])
->filter(fn ($name) => Str::contains($name, $value, ignoreCase: true))
)
プレースホルダテキストとデフォルト値、情報のヒントも指定できます。You may also include placeholder text, a default value, and an informational hint:
$name = suggest(
label: 'What is your name?',
options: ['Taylor', 'Dayle'],
placeholder: 'E.g. Taylor',
default: $user?->name,
hint: 'This will be displayed on your profile.'
);
必須値Required Values
入力値が必須の場合は、required
引数を渡してください。If you require a value to be entered, you may pass the required
argument:
$name = suggest(
label: 'What is your name?',
options: ['Taylor', 'Dayle'],
required: true
);
バリデーション・メッセージをカスタマイズしたい場合は、文字列を渡すこともできます。If you would like to customize the validation message, you may also pass a string:
$name = suggest(
label: 'What is your name?',
options: ['Taylor', 'Dayle'],
required: 'Your name is required.'
);
追加のバリデーションAdditional Validation
最後に、追加のバリデーションロジックを実行したい場合は、validate
引数にクロージャを渡します。Finally, if you would like to perform additional validation logic, you may pass a closure to the validate
argument:
$name = suggest(
label: 'What is your name?',
options: ['Taylor', 'Dayle'],
validate: fn (string $value) => match (true) {
strlen($value) < 3 => 'The name must be at least 3 characters.',
strlen($value) > 255 => 'The name must not exceed 255 characters.',
default => null
}
);
クロージャは入力された値を受け取り、エラーメッセージを返すか、バリデーションに合格した場合は、null
を返します。The closure will receive the value that has been entered and may return an error message, or null
if the validation passes.
検索Search
ユーザーが選択できる選択肢がたくさんある場合、search
機能を使えば、矢印キーを使って選択肢を選択する前に、検索クエリを入力して結果を絞り込むことができます。If you have a lot of options for the user to select from, the search
function allows the user to type a search query to filter the results before using the arrow keys to select an option:
use function Laravel\Prompts\search;
$id = search(
'Search for the user that should receive the mail',
fn (string $value) => strlen($value) > 0
? User::where('name', 'like', "%{$value}%")->pluck('name', 'id')->all()
: []
);
クロージャは、ユーザーがこれまでに入力したテキストを受け取り、 選択肢の配列を返さなければなりません。連想配列を返す場合は選択された選択肢のキーが返され、 そうでない場合はその値が代わりに返されます。The closure will receive the text that has been typed by the user so far and must return an array of options. If you return an associative array then the selected option's key will be returned, otherwise its value will be returned instead.
プレースホルダテキストと情報のヒントも含められます。You may also include placeholder text and an informational hint:
$id = search(
label: 'Search for the user that should receive the mail',
placeholder: 'E.g. Taylor Otwell',
options: fn (string $value) => strlen($value) > 0
? User::where('name', 'like', "%{$value}%")->pluck('name', 'id')->all()
: [],
hint: 'The user will receive an email immediately.'
);
5選択肢を超えると、選択肢がリスト表示されます。scroll
引数を渡し、カスタマイズ可能です。Up to five options will be displayed before the list begins to scroll. You may customize this by passing the scroll
argument:
$id = search(
label: 'Search for the user that should receive the mail',
options: fn (string $value) => strlen($value) > 0
? User::where('name', 'like', "%{$value}%")->pluck('name', 'id')->all()
: [],
scroll: 10
);
バリデーションValidation
追加のバリデーションロジックを実行したい場合は、validate
引数にクロージャを渡します。If you would like to perform additional validation logic, you may pass a closure to the validate
argument:
$id = search(
label: 'Search for the user that should receive the mail',
options: fn (string $value) => strlen($value) > 0
? User::where('name', 'like', "%{$value}%")->pluck('name', 'id')->all()
: [],
validate: function (int|string $value) {
$user = User::findOrFail($value);
if ($user->opted_out) {
return 'This user has opted-out of receiving mail.';
}
}
);
options
引数が連想配列の場合、クロージャは選択されたキーを受け取ります。連想配列でない場合は選択された値を受け取ります。クロージャはエラーメッセージを返すか、バリデーションに成功した場合はnull
を返してください。If the options
closure returns an associative array, then the closure will receive the selected key, otherwise, it will receive the selected value. The closure may return an error message, or null
if the validation passes.
マルチ検索Multi-search
検索可能なオプションがたくさんあり、ユーザーが複数のアイテムを選択できるようにする必要がある場合、multisearch
関数で、ユーザーが矢印キーとスペースバーを使ってオプションを選択する前に、検索クエリを入力してもらい、結果を絞り込めます。If you have a lot of searchable options and need the user to be able to select multiple items, the multisearch
function allows the user to type a search query to filter the results before using the arrow keys and space-bar to select options:
use function Laravel\Prompts\multisearch;
$ids = multisearch(
'Search for the users that should receive the mail',
fn (string $value) => strlen($value) > 0
? User::where('name', 'like', "%{$value}%")->pluck('name', 'id')->all()
: []
);
クロージャは、ユーザーがそれまでにタイプしたテキストを受け取り、オプションの配列を返さなければなりません。クロージャから連想配列を返す場合は、選択済みオプションのキーを返します。それ以外の場合は、代わりに値を返します。The closure will receive the text that has been typed by the user so far and must return an array of options. If you return an associative array then the selected options' keys will be returned; otherwise, their values will be returned instead.
また、プレースホルダテキストと情報ヒントも含められます。You may also include placeholder text and an informational hint:
$ids = multisearch(
label: 'Search for the users that should receive the mail',
placeholder: 'E.g. Taylor Otwell',
options: fn (string $value) => strlen($value) > 0
? User::where('name', 'like', "%{$value}%")->pluck('name', 'id')->all()
: [],
hint: 'The user will receive an email immediately.'
);
リストをスクロールし始める前に、最大5選択肢表示します。scroll
引数を指定し、カスタマイズできます。Up to five options will be displayed before the list begins to scroll. You may customize this by providing the scroll
argument:
$ids = multisearch(
label: 'Search for the users that should receive the mail',
options: fn (string $value) => strlen($value) > 0
? User::where('name', 'like', "%{$value}%")->pluck('name', 'id')->all()
: [],
scroll: 10
);
値の要求Requiring a Value
デフォルトで、ユーザーは0個以上のオプションを選択できます。required
引数を渡せば、1つ以上のオプションを強制できます。By default, the user may select zero or more options. You may pass the required
argument to enforce one or more options instead:
$ids = multisearch(
'Search for the users that should receive the mail',
fn (string $value) => strlen($value) > 0
? User::where('name', 'like', "%{$value}%")->pluck('name', 'id')->all()
: [],
required: true,
);
バリデーションメッセージをカスタマイズする場合は、required
引数へ文字列を指定してください。If you would like to customize the validation message, you may also provide a string to the required
argument:
$ids = multisearch(
'Search for the users that should receive the mail',
fn (string $value) => strlen($value) > 0
? User::where('name', 'like', "%{$value}%")->pluck('name', 'id')->all()
: [],
required: 'You must select at least one user.'
);
バリデーションValidation
バリデーションロジックを追加実行したい場合は、validate
引数にクロージャを渡します。If you would like to perform additional validation logic, you may pass a closure to the validate
argument:
$ids = multisearch(
label: 'Search for the users that should receive the mail',
options: fn (string $value) => strlen($value) > 0
? User::where('name', 'like', "%{$value}%")->pluck('name', 'id')->all()
: [],
validate: function (array $values) {
$optedOut = User::where('name', 'like', '%a%')->findMany($values);
if ($optedOut->isNotEmpty()) {
return $optedOut->pluck('name')->join(', ', ', and ').' have opted out.';
}
}
);
options
クロージャが連想配列を返す場合、クロージャは選択済みのキーを受け取ります。そうでない場合は、選択済みの値を受け取ります。クロージャはエラーメッセージを返すか、バリデーションに合格した場合はnull
を返します。If the options
closure returns an associative array, then the closure will receive the selected keys; otherwise, it will receive the selected values. The closure may return an error message, or null
if the validation passes.
一時停止Pause
pause
関数は、ユーザーへ情報テキストを表示し、Enter/Returnキーが押されるのを待つことにより、ユーザーの続行の意思を確認するために使用します。The pause
function may be used to display informational text to the user and wait for them to confirm their desire to proceed by pressing the Enter / Return key:
use function Laravel\Prompts\pause;
pause('Press ENTER to continue.');
情報メッセージInformational Messages
note
、info
、warning
、error
、alert
関数は、情報メッセージを表示するために使用します。The note
, info
, warning
, error
, and alert
functions may be used to display informational messages:
use function Laravel\Prompts\info;
info('Package installed successfully.');
テーブルTables
table
関数を使うと、複数の行や列のデータを簡単に表示できます。指定する必要があるのは、カラム名とテーブルのデータだけです。The table
function makes it easy to display multiple rows and columns of data. All you need to do is provide the column names and the data for the table:
use function Laravel\Prompts\table;
table(
['Name', 'Email'],
User::all(['name', 'email'])
);
スピンSpin
spin
関数は、指定したコールバックを実行している間、オプションのメッセージとともにスピナーを表示します。これは進行中の処理を示す役割を果たし、完了するとコールバックの結果を返します。The spin
function displays a spinner along with an optional message while executing a specified callback. It serves to indicate ongoing processes and returns the callback's results upon completion:
use function Laravel\Prompts\spin;
$response = spin(
fn () => Http::get('http://example.com'),
'Fetching response...'
);
Warning!
spin
関数でスピナーをアニメーションするために、pcntl
PHP拡張モジュールが必要です。この拡張モジュールが利用できない場合は、代わりに静的なスピナーが表示されます。[!WARNING]
Thespin
function requires thepcntl
PHP extension to animate the spinner. When this extension is not available, a static version of the spinner will appear instead.
プログレスバーProgress Bars
実行に長時間かかるタスクの場合、タスクの完了度をユーザーに知らせるプログレスバーを表示すると便利です。progress
関数を使用すると、Laravelはプログレスバーを表示し、指定する反復可能な値を繰り返し処理するごとに進捗を進めます。For long running tasks, it can be helpful to show a progress bar that informs users how complete the task is. Using the progress
function, Laravel will display a progress bar and advance its progress for each iteration over a given iterable value:
use function Laravel\Prompts\progress;
$users = progress(
label: 'Updating users',
steps: User::all(),
callback: fn ($user) => $this->performTask($user),
);
progress
関数はマップ関数のように動作し、コールバックの各繰り返しの戻り値を含む配列を返します。The progress
function acts like a map function and will return an array containing the return value of each iteration of your callback.
このコールバックは、\Laravel\Prompts\Progress
インスタンスも受け取り可能で、繰り返しごとにラベルとヒントを修正できます。The callback may also accept the \Laravel\Prompts\Progress
instance, allowing you to modify the label and hint on each iteration:
$users = progress(
label: 'Updating users',
steps: User::all(),
callback: function ($user, $progress) {
$progress
->label("Updating {$user->name}")
->hint("Created on {$user->created_at}");
return $this->performTask($user);
},
hint: 'This may take some time.',
);
プログレス・バーの進め方を手作業でコントロールする必要がある場合があります。まず、プロセスが反復処理するステップの総数を定義します。そして、各アイテムを処理した後にadvance
メソッドでプログレスバーを進めます。Sometimes, you may need more manual control over how a progress bar is advanced. First, define the total number of steps the process will iterate through. Then, advance the progress bar via the advance
method after processing each item:
$progress = progress(label: 'Updating users', steps: 10);
$users = User::all();
$progress->start();
foreach ($users as $user) {
$this->performTask($user);
$progress->advance();
}
$progress->finish();
ターミナルの考察Terminal Considerations
ターミナルの横幅Terminal Width
ラベルやオプション、バリデーションメッセージの長さが、ユーザーの端末の「列」の文字数を超える場合、自動的に切り捨てます。ユーザーが狭い端末を使う可能性がある場合は、これらの文字列の長さを最小限にする検討をしてください。一般的に安全な最大長は、80文字の端末をサポートする場合、74文字です。If the length of any label, option, or validation message exceeds the number of "columns" in the user's terminal, it will be automatically truncated to fit. Consider minimizing the length of these strings if your users may be using narrower terminals. A typically safe maximum length is 74 characters to support an 80-character terminal.
ターミナルの高さTerminal Height
scroll
引数を受け入れるプロンプトの場合、設定済み値は、検証メッセージ用のスペースを含めて、ユーザーの端末の高さに合わせて自動的に縮小されます。For any prompts that accept the scroll
argument, the configured value will automatically be reduced to fit the height of the user's terminal, including space for a validation message.
未サポートの環境とフォールバックUnsupported Environments and Fallbacks
Laravel PromptsはmacOS、Linux、WindowsのWSLをサポートしています。Windows版のPHPの制限により、現在のところWSL以外のWindowsでは、Laravel Promptsを使用できません。Laravel Prompts supports macOS, Linux, and Windows with WSL. Due to limitations in the Windows version of PHP, it is not currently possible to use Laravel Prompts on Windows outside of WSL.
このため、Laravel PromptsはSymfony Console Question Helperのような代替実装へのフォールバックをサポートしています。For this reason, Laravel Prompts supports falling back to an alternative implementation such as the Symfony Console Question Helper[https://symfony.com/doc/current/components/console/helpers/questionhelper.html].
[!NOTE]
Note: LaravelフレームワークでLaravel Promptsを使用する場合、各プロンプトのフォールバックが設定済みで、未サポートの環境では自動的に有効になります。
When using Laravel Prompts with the Laravel framework, fallbacks for each prompt have been configured for you and will be automatically enabled in unsupported environments.
フォールバックの条件Fallback Conditions
Laravelを使用していない場合や、フォールバック動作をカスタマイズする必要がある場合は、Prompt
クラスのfallbackWhen
へブール値を渡してください。If you are not using Laravel or need to customize when the fallback behavior is used, you may pass a boolean to the fallbackWhen
static method on the Prompt
class:
use Laravel\Prompts\Prompt;
Prompt::fallbackWhen(
! $input->isInteractive() || windows_os() || app()->runningUnitTests()
);
フォールバックの振る舞いFallback Behavior
Laravelを使用していない場合や、フォールバックの動作をカスタマイズする必要がある場合は、各プロンプトクラスのfallbackUsing
静的メソッドへクロージャを渡してください。If you are not using Laravel or need to customize the fallback behavior, you may pass a closure to the fallbackUsing
static method on each prompt class:
use Laravel\Prompts\TextPrompt;
use Symfony\Component\Console\Question\Question;
use Symfony\Component\Console\Style\SymfonyStyle;
TextPrompt::fallbackUsing(function (TextPrompt $prompt) use ($input, $output) {
$question = (new Question($prompt->label, $prompt->default ?: null))
->setValidator(function ($answer) use ($prompt) {
if ($prompt->required && $answer === null) {
throw new \RuntimeException(is_string($prompt->required) ? $prompt->required : 'Required.');
}
if ($prompt->validate) {
$error = ($prompt->validate)($answer ?? '');
if ($error) {
throw new \RuntimeException($error);
}
}
return $answer;
});
return (new SymfonyStyle($input, $output))
->askQuestion($question);
});
フォールバックは、プロンプトクラスごとに個別に設定する必要があります。クロージャはプロンプトクラスのインスタンスを受け取り、 プロンプトの適切な型を返す必要があります。Fallbacks must be configured individually for each prompt class. The closure will receive an instance of the prompt class and must return an appropriate type for the prompt.